C programming on esieabot
pigpiod
To program on the esieabot, we use pigpiod. pigpio is a library for controlling GPIOs. Unlike WiringPi, it is still maintained to this day. pigpiod is a way of using pigpio with a central service allowing several programs to be launched at once, without monopolizing the GPIOs.
This documentation is a condensed version of the official pigpiod documentation <http://abyz.me.uk/rpi/pigpio/pdif2.html>`_.
Compiling a program with pigpiod
gcc fichier.c -o executable -lpigpiod_if2
To compile a program using the pigpiod library, you need to add the -lpigpiod_if2 option to your gcc command.
#include <pigpiod_if2.h>
All that’s left is to add the library header to your code.
Basics
When you run a program using pigpiod, it communicates with the pigpiod service in order to transmit its commands. Before launching a program, make sure that the service is running with the command systemctl status pigpiod
. Several programs may be running at the same time. If they send contradictory commands, pigpiod will only take into account the last command received.
As on an Arduino, there are a multitude of GPIO pins that can be used. More information can be found in the Raspberry Pi documentation. A number of GPIOs are already in use on your esieabot for basic functions. Refer to your esieabot’s assembly manual.
As on an Arduino, GPIOs can be used as input or output, to receive or send logic signals. On a Raspberry Pi, logic signals must be at 3.3V, not 5V. In all cases, at the start of the program, initialize the connection to the pigpiod service with this function :
int pi = pigpio_start(NULL, NULL);
if (pi < 0) {
printf("Can't connect to pigpiod");
exit(-1);
}
The pi variable then represents the esieabot entity. To interrupt the connection with the pigpiod service, use the following function at the end of the program:
pigpio_stop(pi);
Note
In the following examples, include unistd.h
to use the sleep()
function.
GPIO “Hello World!”: light an LED
First, connect the LED to an unused GPIO, for example GPIO 16. Don’t forget to connect a resistor in series to limit the current.
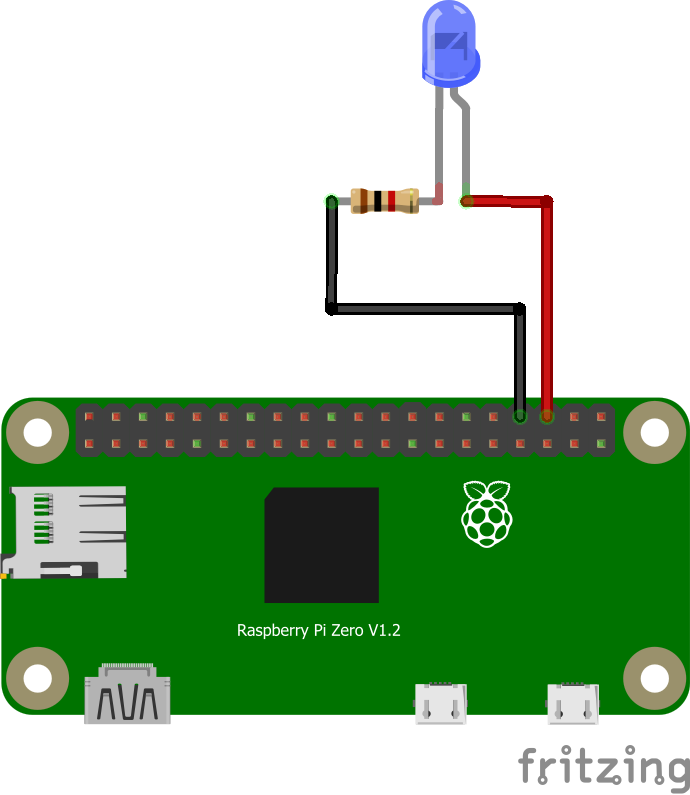
Next, define GPIO 16 as an output with the following function :
set_mode(pi, 16, PI_OUTPUT); // Set GPIO 16 to output mode
Finally, you can turn it on and off with this simple loop :
while(1) {
gpio_write(pi, 16, PI_HIGH); // Send a "HIGH" signal to GPIO 16 to turn it on
sleep(1);
gpio_write(pi, 16, PI_LOW); // Send a "LOW" signal to GPIO16 to turn it off
sleep(1);
}
If everything’s connected correctly, you should have an LED flashing every second!
Using an H-bridge
The commands for using an H-bridge are the same as for controlling an LED, since the latter only needs to receive logic signals to switch a motor on and off. Refer to documentation of the H-bridge and assembly manual for the signals to be sent.
Using a servomotor
To use a servomotor, you need to send signals of very precise duration to communicate an angle setpoint. More information on servomotor documentation. With the pigpiod library, you can transmit an angle setpoint directly to a servomotor, without having to worry about the precise signals. Setpoints range from 500 to 2500. 0 disarms the servomotor. Any GPIO can be used as a signal source. For greater precision (to avoid “jitter” effects) it is possible to use the hardware PWM ports of Raspberry Pi.
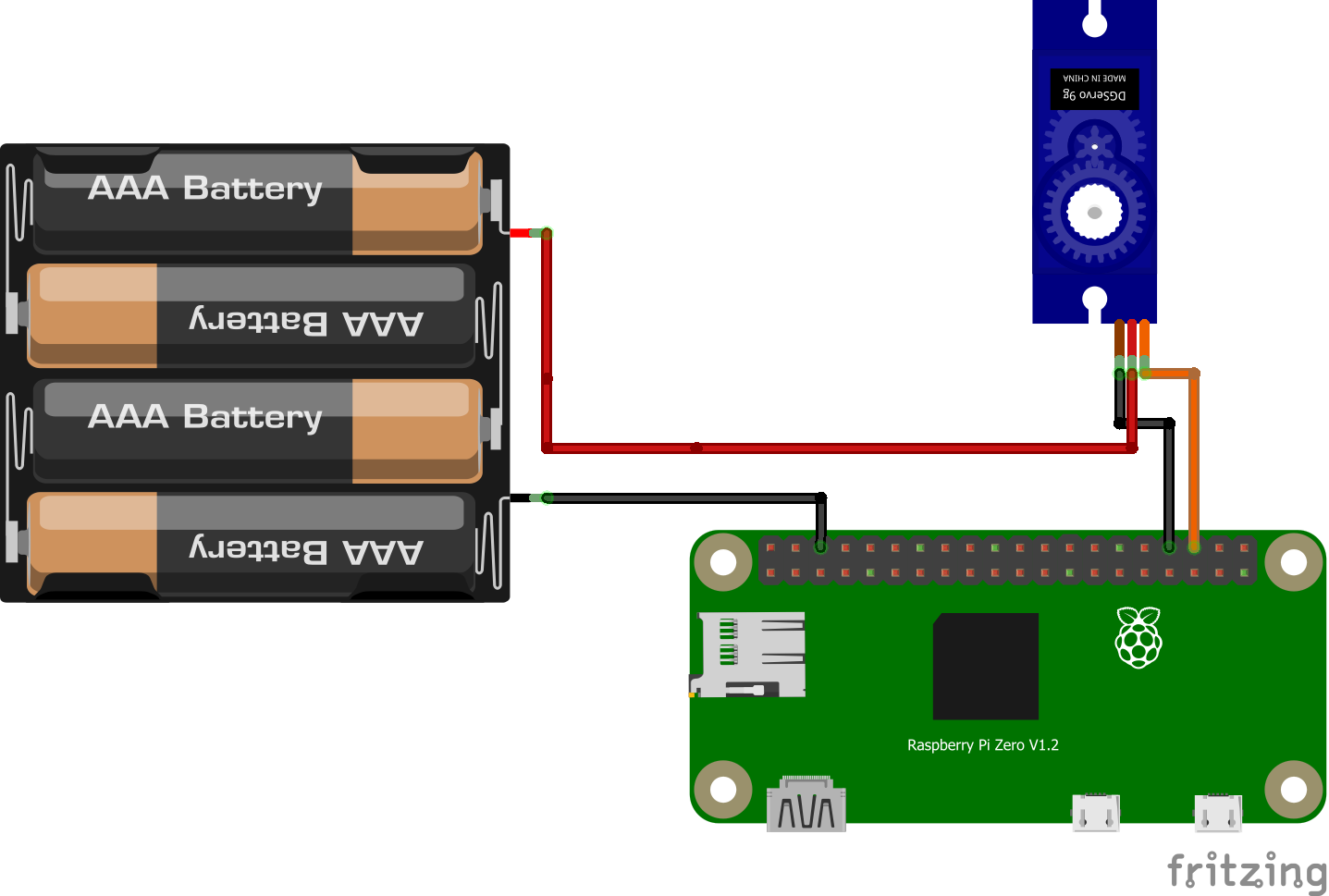
set_mode(pi, 16, PI_OUTPUT); // Set GPIO 16 to output mode
set_servo_pulsewidth(pi, 16, 500); // Set servo motor to minimum value
sleep(1);
set_servo_pulsewidth(pi, 16, 1500); // Set servo motor to middle position
sleep(1);
set_servo_pulsewidth(pi, 16, 2500); // Set servo motor to maximum position
sleep(1);
set_servo_pulsewidth(pi, 16, 0); // Disarms servo motor
Sending a PWM signal
A PWM (Pulse Width Modulation) signal is used to control an electronic component as if it were controlled by an analog signal that varies in voltage. To do this, we’ll rapidly transmit HIGH and LOW signals with a bandwidth that varies according to the desired average voltage. With a Raspberry Pi, all GPIOs can be used to emit this kind of signal. In concrete terms, this makes it possible to vary the brightness of an LED or the speed of a DC motor.
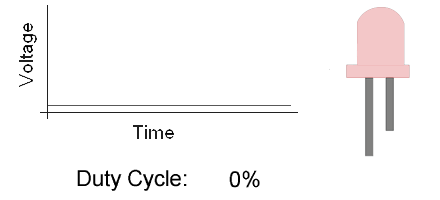
Illustration PyroElectro, http://www.pyroelectro.com/tutorials/fading_led_pwm/theory.html
With pigpiod, we use the set_PWM_dutycycle()
function to emit a PWM signal. The requested bandwidth must be between 0 and 255. 0 corresponds to off, 255 to on.
set_mode(pi, 16, PI_OUTPUT); // Set GPIO 16 to output mode
set_PWM_dutycycle(pi, 16, 0); // Produces an average voltage of 0V (as if sending a LOW signal)
sleep(1);
set_PWM_dutycycle(pi, 16, 128); // Produces an average voltage of 1.65V (half of 3.3V)
sleep(1);
set_PWM_dutycycle(pi, 16, 255); // Produces an average voltage of 3.3V (as if sending a HIGH signal)